JSONpath
JSON Query Language "JSONPath" has been used to extract the parts of a given document. It is to its family JSON the same as an XPATH to an XML. And this is applicable in many programming languages like Java, JavaScript, Python, and PHP.
It is used in API AlertSite for endpoint monitors and this JSONPath layout of the JSON fields needs to be verified. For those processes, It grants us to compile a JSONPath string to use it multiple times or to compile and apply it in one major single demand operation.
Hint sentence to keep in mind - "It is mainly used for activity selecting and extracting of the subsection of the JSON document".
JSONPath expressions cover properties like names and values, those two are Case-sensitive. And It is the same as an XPath because these two do not have an operation to access the parent or sibling nodes from the provided node.
JSON Path phrase stipulates the path to a set of elements in JSON structures. And it can use or value both dot notation and bracket notation or else vice versa. Hint note for the user (Dots are only used before the property names, not inside the brackets).
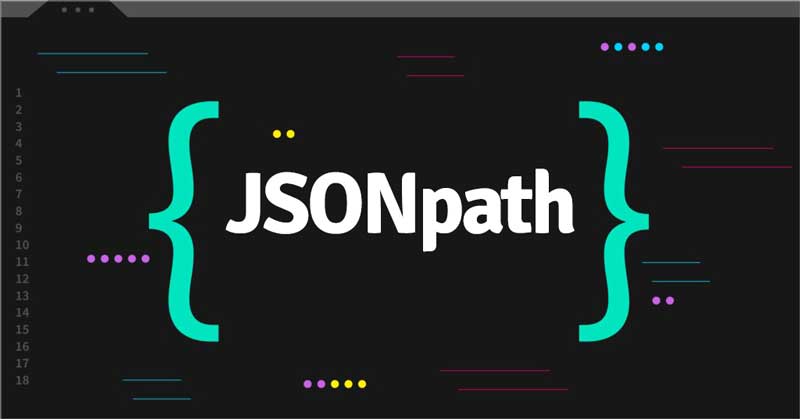
JSONpath Notation:
- Dot notation - Ex:- $.shop.product[0].title
- Bracket notation - EX:- $[‘shop’][‘product’][0][‘title’]
- Mingle of dot and bracket notation - Ex:- $[‘shop’].product[0].title
JSONpath Syntax Elements:
Elements | Explanation |
---|---|
$ | It represents the root object or array of elements and it would be the neglectable expression. |
.property | It selects the selective property in a parent object. |
[‘property’] | It’s another representation of property expression with the bracket notation. |
[n] | It selects an n-th element from an array elements group and its index value is 0-based. |
[index1, index2, …] | It selects an array of elements with the specified index values and returns a list. |
..property | It uses the Recursive Descent Parsing method to search for the specified property name recursively and returns an array of values with the property name specifically. And one more asset of this property expression is it returns a list, even if just one property is found in this recursive search. |
Star symbol * | It selects all elements in an object or an array of elements, with a blind note of names or indexes. It denotes or layout the items inside the object. |
[start:end] | [start:] - It selects the array elements from the start index value and up to, but not the end index value. If we have not mentioned this end in this expression it selects all the elements from start to end of the array list. And it returns the list successfully. |
[:n] | It returns the list with the action of selecting the first n elements of the array. |
[-n:] | It returns the list with the action of selecting the last n elements of the array. |
[?(expression)] | It selects all elements in an object or array list that match the stated or specified filter. And it returns a list called a Filter expression. |
[(expression)] | It’s a script expression and it can be used in place of explicit property names or indexes. Let’s see with one example [(@.length-1)] by this expression we will select the last items of an array. JSON field named length here and it represents the length of the current array. |
@ | It is used in a filter expression to hint at the current node being processed. |
JSONpath Examples
key selector examples
{
"id": 1,
"yourName": "Azar",
"sec": {
"box": "smk"
}
}
- selector: .yourName
result: "Azar" - selector: .sec.box
result: "smk"
key selector with dot in name
{ "name.smk": 22 }
- selector: name.smk
result: "22"
key selector with space in name
{ "name smk": 22 }
- selector: name smk
result: "22"
JSONpath array indexing
[
{
"id": 1,
"yourName": "Peter",
}
]
- selector: [0].id
result: 1
JSONpath array length
{ "clients": [1,2,3,4,5] }
- selector: .clients.length()
result: 5
JSONpath object length
{ "boxs": {"box1": 1, "box2": 2} }
- selector: .boxs.length()
result: 2
String length
{ "name": "peter" }
- selector: .name.length()
result: 5
JSONPath wildcard syntax examples
array wildcard example
{
"books": [
{
"order": 1,
"description": "Article"
},
{
"order": 2,
"description": "Blog",
"introduction": "index"
}
]
}
- selector: .books[].introduction
result: "index" - selector: .books[].order
result: Cannot select JSON value. Selecting multiple nodes is not supported.
object wildcard example
{
"book": {
"id": 1,
"data": "smk"
},
"store": {
"id": 1,
"data": "index",
"books": "Articles"
},
"blog": [
{
"step_num": 1,
"define": "content"
}
]
}
- selector: [*].books
result: "Articles" - selector: [].books
result: "Articles" - selector: [].data
result: Cannot select JSON value. Selecting multiple nodes is not supported.
This Queries that returns multiable elements - Example Below:
JSON Path expression can also return multiable elements. For example, given below:
{
"book": "type of flowers",
"bookCode": [
{
"type": "nature",
"number": "333-999"
},
{
"type": "job",
"number": "666-777"
}
]
}
JSONpath Syntax
bookCode[*].number
It returns the containing two book numbers:
[333-999, 666-777]